7)Python Program to Display the multiplication Table
In Python, you can make a program to display the multiplication table of any number. The following program displays the multiplication table (from 1 to 10) according to the user input.
The following example shows the multiplication table of 18.
See this example:

num = int(input("Show the multiplication table of? "))
# using for loop to iterate multiplication 10 times
for i in range(1,11):
print(num,'x',i,'=',num*i)
8) Python Program to Print the Fibonacci sequence
Fibonacci sequence:
The Fibonacci sequence specifies a series of numbers where the next number is found by adding up the two numbers just before it.
For example:
-
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on....
See this example:
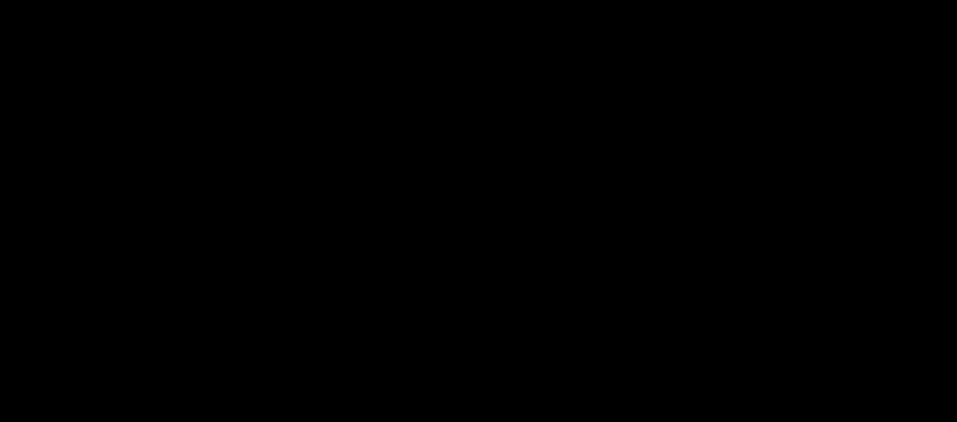
nterms = int(input("How many terms you want? "))
# first two terms
n1 = 0
n2 = 1
count = 2
# check if the number of terms is valid
if nterms <= 0:
print("Plese enter a positive integer")
elif nterms == 1:
print("Fibonacci sequence:")
print(n1)
else:
print("Fibonacci sequence:")
print(n1,",",n2,end=', ')
while count < nterms:
nth = n1 + n2
print(nth,end=' , ')
# update values
n1 = n2
n2 = nth
count += 1