9)Python Program to Check Armstrong Number
Armstrong number:
A number is called Armstrong number if it is equal to the sum of the cubes of its own digits.
example: 153 is an Armstrong number since 153 = 1*1*1 + 5*5*5 + 3*3*3.
The Armstrong number is also known as narcissistic number.
See this example:

num = int(input("Enter a number: "))
sum = 0
temp = num
while temp > 0:
digit = temp % 10
sum += digit ** 3
temp //= 10
if num == sum:
print(num,"is an Armstrong number")
else:
print(num,"is not an Armstrong number")
10) Python Program to Find Armstrong Number between an Interval
We have already read the concept of Armstrong numbers in the previous program. Here, we print the Armstrong numbers within a specific given interval.
This example shows all Armstrong numbers between 100 and 500.
See this example:
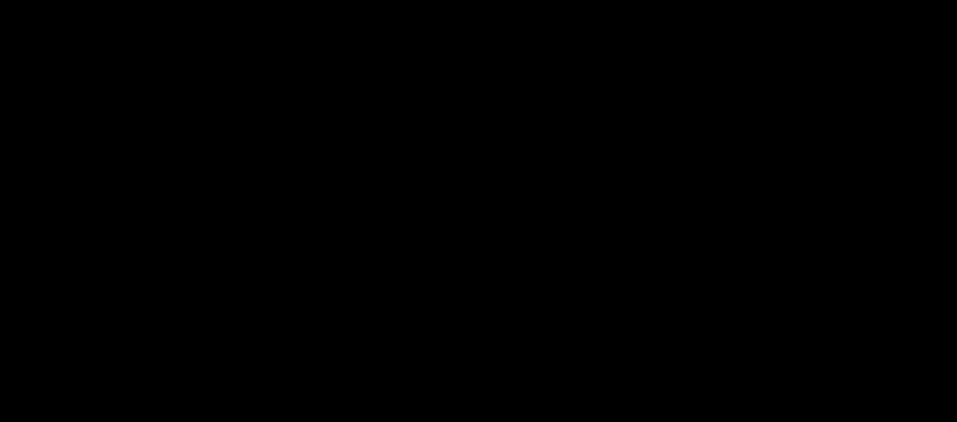
lower = int(input("Enter lower range: "))
upper = int(input("Enter upper range: "))
for num in range(lower,upper + 1):
sum = 0
temp = num
while temp > 0:
digit = temp % 10
sum += digit ** 3
temp //= 10
if num == sum:
print(num)