5)Python Program to Print all Prime Numbers between an Interval
We have already read the concept of prime numbers in the previous program. Here, we are going to print the prime numbers between given interval.
This example will show the prime numbers between 10 and 50.
See this example:

#Take the input from the user:
lower = int(input("Enter lower range: "))
upper = int(input("Enter upper range: "))
for num in range(lower,upper + 1):
if num > 1:
for i in range(2,num):
if (num % i) == 0:
break
else:
print(num)
6) Python Program to Find the Factorial of a Number
What is factorial?
Factorial is a non-negative integer. It is the product of all positive integers less than or equal to that number for which you ask for factorial. It is denoted by exclamation sign (!).
For example:
4! = 4x3x2x1 = 24
The factorial value of 4 is 24.
Note: The factorial value of 0 is 1 always. (Rule violation)
The following example displays the factorial of 5 and -5.
See this example:
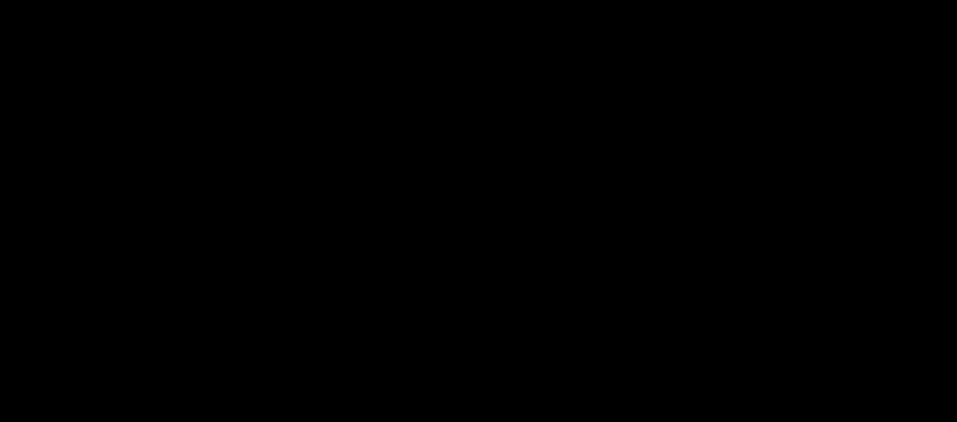
num = int(input("Enter a number: "))
factorial = 1
if num < 0:
print("Sorry, factorial does not exist for negative numbers")
elif num == 0:
print("The factorial of 0 is 1")
else:
for i in range(1,num + 1):
factorial = factorial*i
print("The factorial of",num,"is",factorial)