3) Python Program to Convert Decimal to Binary, Octal and Hexadecimal
Decimal System:
The most widely used number system is decimal system. This system is base 10 number system. In this system, ten numbers (0-9) are used to represent a number.
Binary System: Binary system is base 2 number system. Binary system is used because computers only understand binary numbers (0 and 1).
Octal System:
Octal system is base 8 number system.
Hexadecimal System:
Hexadecimal system is base 16 number system.
This program is written to convert decimal to binary, octal and hexadecimal.
See this example:

dec = int(input("Enter a decimal number: "))
print(bin(dec),"in binary.")
print(oct(dec),"in octal.")
print(hex(dec),"in hexadecimal."
4)Python Program To Find ASCII value of a character
ASCII:
ASCII is an acronym stands for American Standard Code for Information Interchange. In ASCII, a specific numerical value is given to different characters and symbols, for computers to store and manipulate.
It is case sensitive. Same character, having different format (upper case and lower case) has different value. For example: The ASCII value of "A" is 65 while the ASCII value of "a" is 97.
See this example:
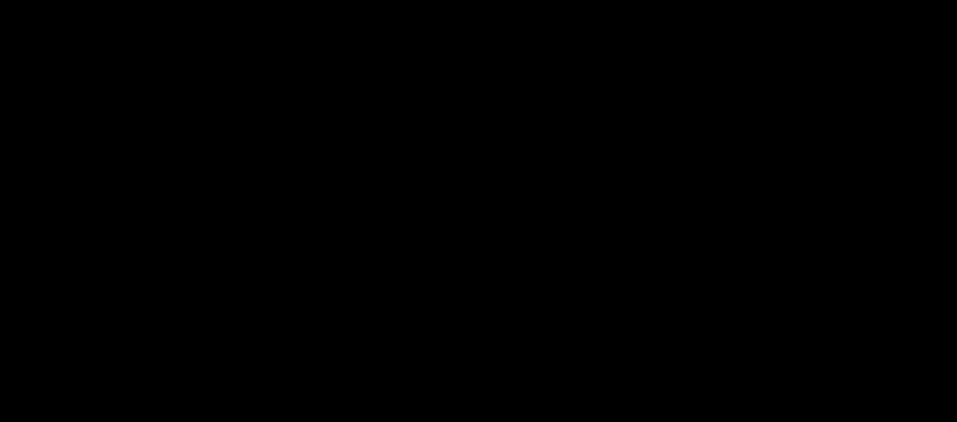
c = input("Enter a character: ")
print("The ASCII value of '" + c + "' is",ord(c))