Python Function Programs
1) Python Program to Find LCM
LCM: Least Common Multiple/ Lowest Common Multiple:
LCM stands for Least Common Multiple. It is a concept of arithmetic and number system. The LCM of two integers a and b is denoted by LCM (a,b). It is the smallest positive integer that is divisible by both "a" and "b".
For example: We have two integers 4 and 6. Let's find LCM
Multiples of 4 are:
-
4, 8, 12, 16, 20, 24, 28, 32, 36,... and so on...
Multiples of 6 are:
-
6, 12, 18, 24, 30, 36, 42,... and so on....
Common multiples of 4 and 6 are simply the numbers that are in both lists:
-
12, 24, 36, 48, 60, 72,.... and so on....
LCM is the lowest common multiplier so it is 12.
See this example:

def lcm(x, y):
if x > y:
greater = x
else:
greater = y
while(True):
if((greater % x == 0) and (greater % y == 0)):
lcm = greater
break
greater += 1
return lcm
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
print("The L.C.M. of", num1,"and", num2,"is", lcm(num1, num2))
2) Python Program to Find HCF
HCF: Highest Common Factor
Highest Common Factor or Greatest Common Divisor of two or more integers when at least one of them is not zero is the largest positive integer that evenly divides the numbers without a remainder. For example, the GCD of 8 and 12 is 4.
For example:
We have two integers 8 and 12. Let's find the HCF.
The divisors of 8 are:
-
1, 2, 4, 8
The divisors of 12 are:
-
1, 2, 3, 4, 6, 12
HCF /GCD is the greatest common divisor. So HCF of 8 and 12 are 4.
See this example:
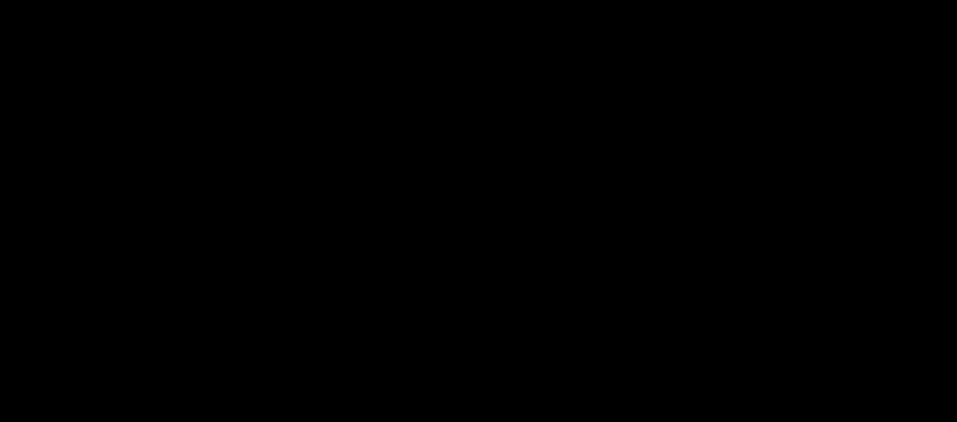
def hcf(x, y):
if x > y:
smaller = y
else:
smaller = x
for i in range(1,smaller + 1):
if((x % i == 0) and (y % i == 0)):
hcf = i
return hcf
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
print("The H.C.F. of", num1,"and", num2,"is", hcf(num1, num2))