5) Python Program to Make a Simple Calculator
In Python, you can create a simple calculator, displaying the different arithmetical operations i.e. addition, subtraction, multiplication and division.
The following program is intended to write a simple calculator in Python:
See this example:

# define functions
def add(x, y):
"""This function adds two numbers""
return x + y
def subtract(x, y):
"""This function subtracts two numbers"""
return x - y
def multiply(x, y):
"""This function multiplies two numbers"""
return x * y
def divide(x, y):
"""This function divides two numbers"""
return x / y
# take input from the user
print("Select operation.")
print("1.Add")
print("2.Subtract")

print("3.Multiply")
print("4.Divide")
choice = input("Enter choice(1/2/3/4):")
num1 = int(input("Enter first number: "))
num2 = int(input("Enter second number: "))
if choice == '1':
print(num1,"+",num2,"=", add(num1,num2))
elif choice == '2':
print(num1,"-",num2,"=", subtract(num1,num2))
elif choice == '3':
print(num1,"*",num2,"=", multiply(num1,num2))
elif choice == '4':
print(num1,"/",num2,"=", divide(num1,num2))
else:
print("Invalid input")
6)Python Function to Display Calendar
In Python, we can display the calendar of any month of any year by importing the calendar module.
See this example:
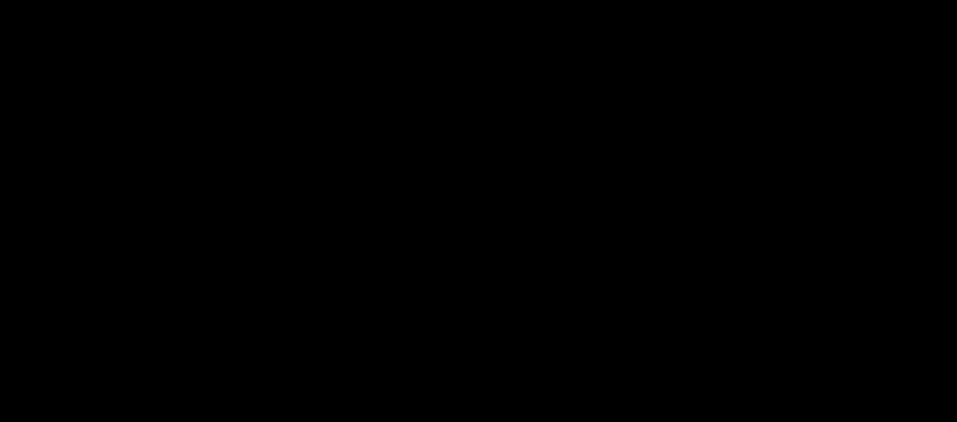
# First import the calendar module
import calendar
# ask of month and year
yy = int(input("Enter year: "))
mm = int(input("Enter month: "))
# display the calendar
print(calendar.month(yy,mm))