13) Decimal to Binary
We can convert any decimal number (base-10 (0 to 9)) into binary number(base-2 (0 or 1)) by c program.
Decimal Number
Decimal number is a base 10 number because it ranges from 0 to 9, there are total 10 digits between 0 to 9. Any combination of digits is decimal number such as 23, 445, 132, 0, 2 etc.
Binary Number
Binary number is a base 2 number because it is either 0 or 1. Any combination of 0 and 1 is binary number such as 1001, 101, 11111, 101010 etc.
Let's see the some binary numbers for the decimal number.
Decimal to Binary Conversion Algorithm
-
Step 1: Divide the number by 2 through % (modulus operator) and store the remainder in array
-
Step 2: Divide the number by 2 through / (division operator)
-
Step 3: Repeat the step 2 until number is greater than 0
Let's see the c example to convert decimal to binary.
Write a c program to convert decimal number to binary.
Input: 5
Output: 101
Input: 20
Output: 10100
Let's see the c example to convert decimal to binary.
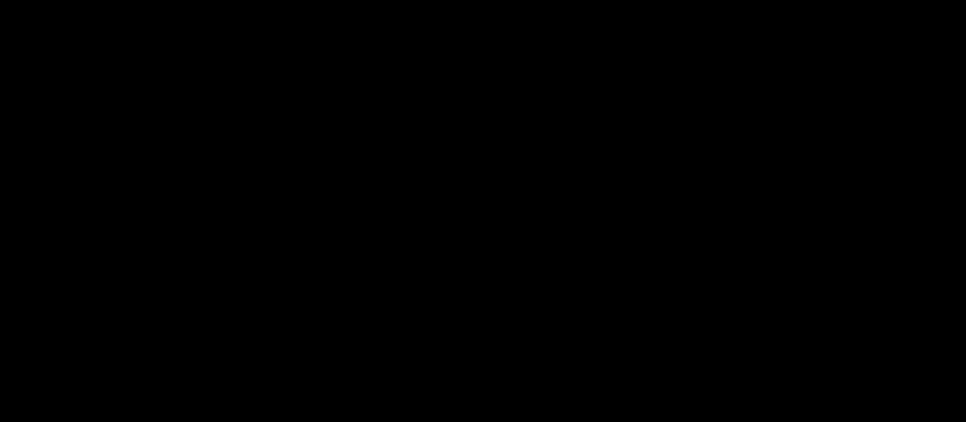
#include<stdio.h>
#include<stdlib.h>
int main(){
int a[10],n,i;
system ("cls");
printf("Enter the number to convert: ");
scanf("%d",&n);
for(i=0;n>0;i++)
{
a[i]=n%2;
n=n/2;
}
printf("\nBinary of Given Number is=");
for(i=i-1;i>=0;i--)
{
printf("%d",a[i]);
}
return 0;
}
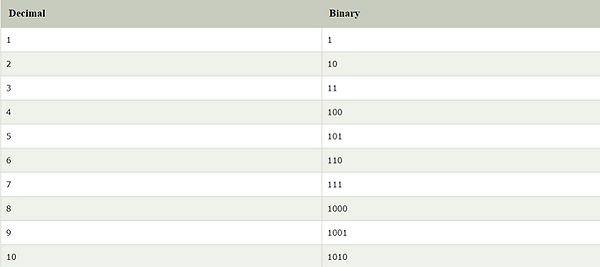
14) Alphabet Triangle
There are different triangles that can be printed. Triangles can be generated by alphabets or numbers. In this c program, we are going to print alphabet triangles.
Let's see the c example to print alphabet triangle.
Write a c program to print alphabet triangle.
Output:
A ABA ABCBA ABCDCBA ABCDEDCBA
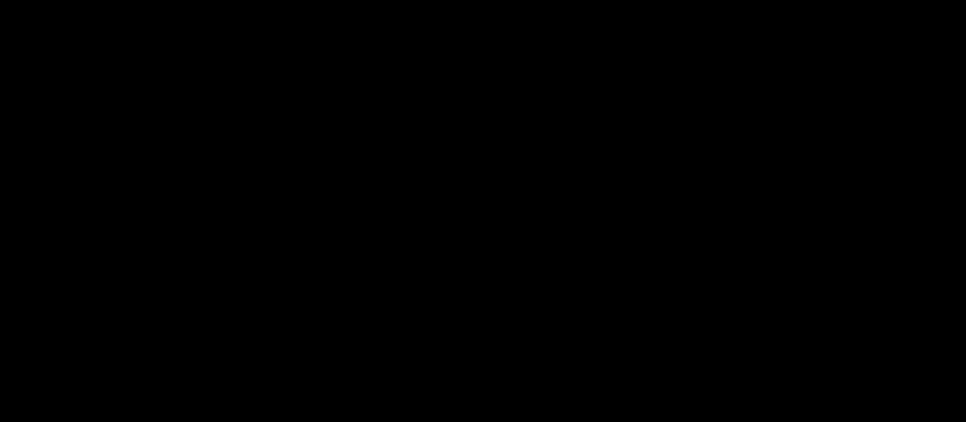
#include<stdio.h>
#include<stdlib.h>
int main(){
int ch=65;
int i,j,k,m;
system("cls");
for(i=1;i<=5;i++)
{
for(j=5;j>=i;j--)
printf(" ");
for(k=1;k<=i;k++)
printf("%c",ch++);
ch--;
for(m=1;m<i;m++)
printf("%c",--ch);
printf("\n");
ch=65;
}
return 0;
}
15) Number Triangle
Like alphabet triangle, we can write the c program to print the number triangle. The number triangle can be printed in different ways.
Let's see the c example to print number triangle.
Write a c program to print number triangle.
Input: 7
Output:
enter the range= 6 1 121 12321 1234321 123454321 12345654321
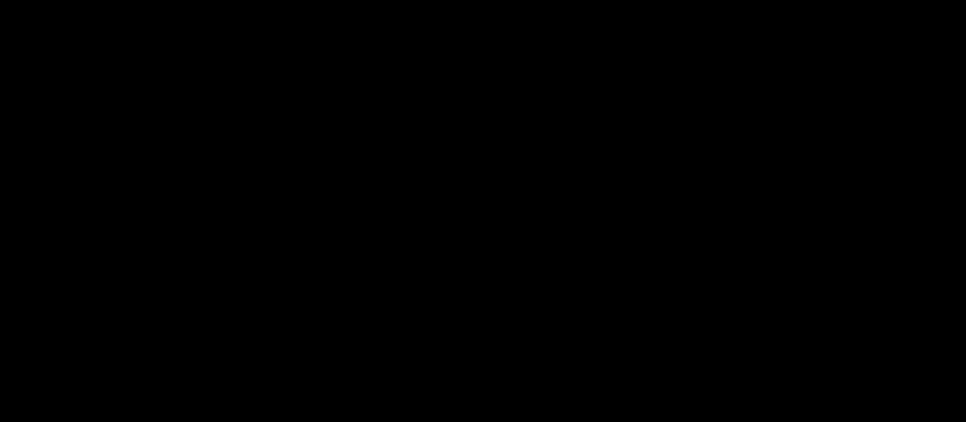
#include<stdio.h>
#include<stdlib.h>
int main(){
int i,j,k,l,n;
system("cls");
printf("enter the range=");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(j=1;j<=n-i;j++)
{
printf(" ");
}
for(k=1;k<=i;k++)
{
printf("%d",k);
}
for(l=i-1;l>=1;l--)
{
printf("%d",l);
}
printf("\n");
}
return 0;
}