16) Fibonacci Triangle
In this program, we are getting input from the user for the limit for fibonacci triangle, and printing the fibonacci series for the given number of times (limit).
Let's see the c example to generate fibonacci triangle.
Write a c program to generate fibonacci triangle.
Input: 5
Output:
1 1 1 1 1 2 1 1 2 3 1 1 2 3 5
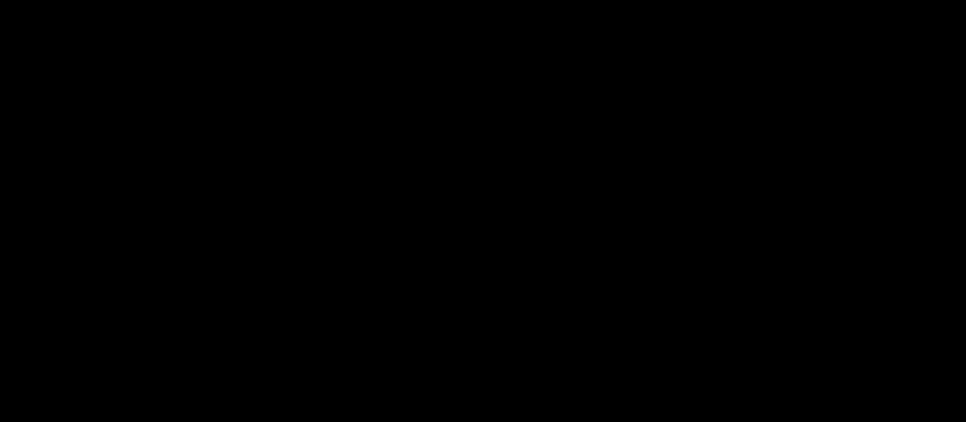
#include<stdio.h>
#include<stdlib.h>
int main(){
int a=0,b=1,i,c,n,j;
system("cls");
printf("Enter the limit:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
a=0;
b=1;
printf("%d\t",b);
for(j=1;j<i;j++)
{
c=a+b;
printf("%d\t",c);
a=b;
b=c;
}
printf("\n");
}
return 0;
}
17) Number in Characters
In c language, we can easily convert number in characters by the help of loop and switch case. In this program, we are taking input from the user and iterating this number until it is 0. While iteration, we are dividing it by 10 and the remainder is passed in switch case to get the word for the number.
Write a c program to convert number in characters.
Input: 5
Output: five
Input: 203
Output: two zero three
Let's see the c program to convert number in characters.
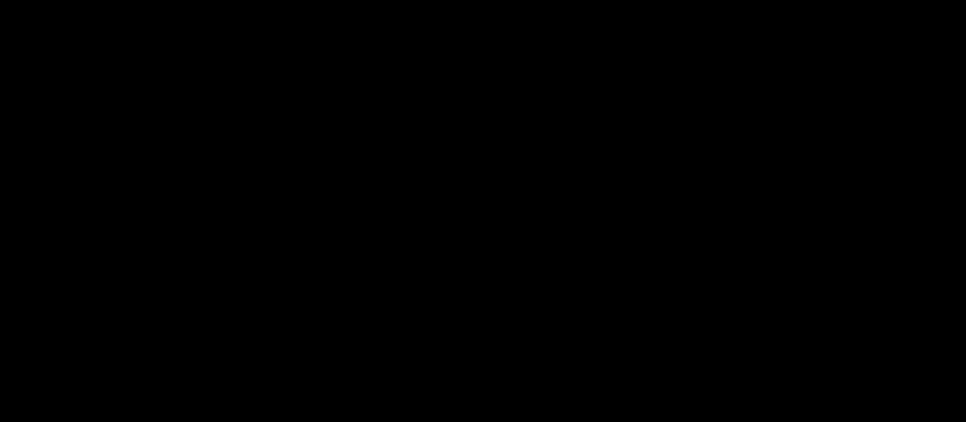
#include<stdio.h>
#include<stdlib.h>
int main(){
long int n,sum=0,r;
system("cls");
printf("enter the number=");
scanf("%ld",&n);
while(n>0)
{
r=n%10;
sum=sum*10+r;
n=n/10;
}
n=sum;
while(n>0)
{
r=n%10;
switch(r)
{
case 1:
printf("one ");
break;
case 2:
printf("two ");
break;
case 3:
printf("three ");
break;
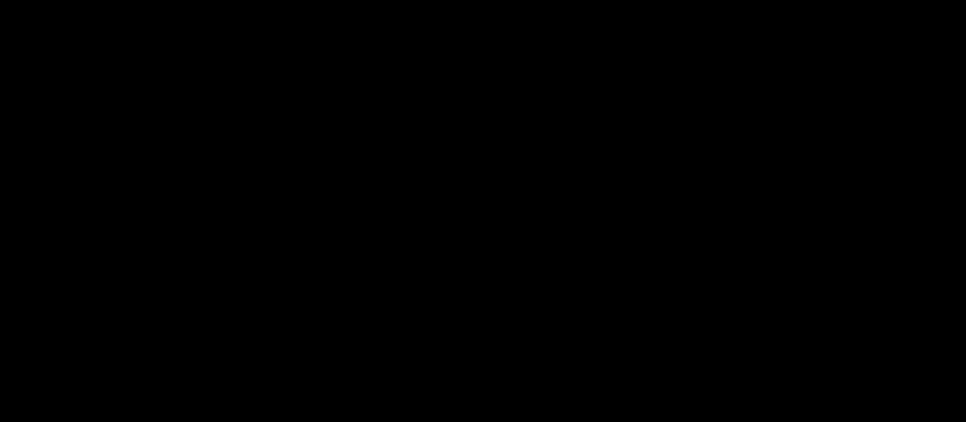
case 4:
printf("four ");
break;
case 5:
printf("five ");
break;
case 6:
printf("six ");
break;
case 7:
printf("seven ");
break;
case 8:
printf("eight ");
break;
case 9:
printf("nine ");
break;
case 0:
printf("zero ");
break;
default:
printf("tttt");
break;
}
n=n/10;
}
return 0;
}