10) Assembly Program in C
We can write assembly program code inside c language program. In such case, all the assembly code must be placed inside asm{} block.
Let's see a simple assembly program code to add two numbers in c program.
Write a c program to add two numbers using assembly code.
Note: We have executed this program on TurboC.
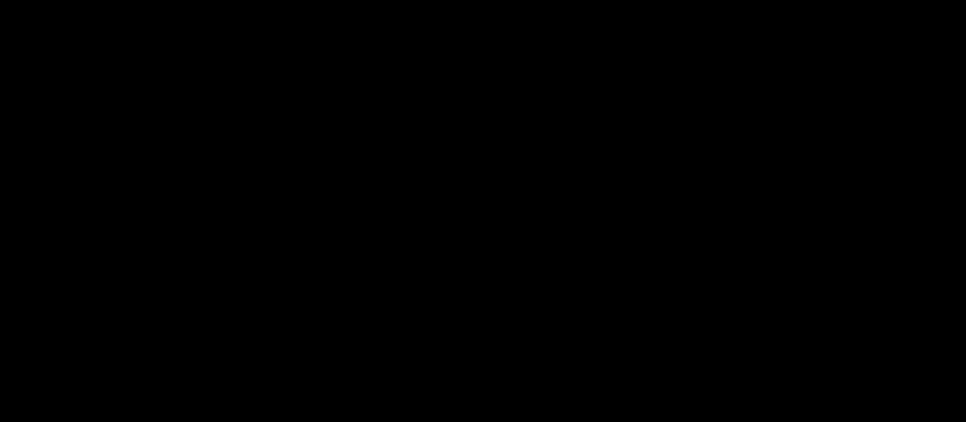
#include<stdio.h>
void main() {
int a = 10, b = 20, c;
asm {
mov ax,a
mov bx,b
add ax,bx
mov c,ax
}
printf("c= %d",c);
}
11) C Program without main() function
We can write c program without using main() function. To do so, we need to use #define preprocessor directive.
Let's see a simple program to print "hello" without main() function.
Write a c program to print "Hello" without using main() function.
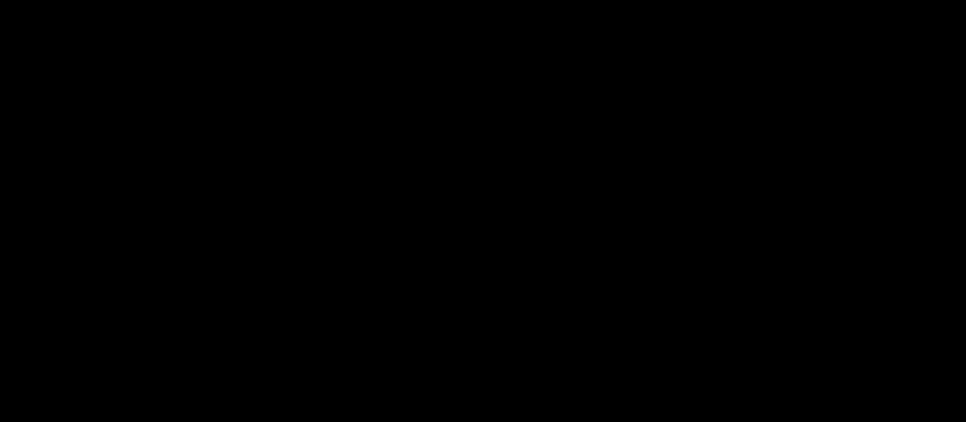
#include<stdio.h>
#define start main
void start() {
printf("Hello");
}
12) Matrix Multiplication
We can add, subtract, multiply and divide 2 matrices. To do so, we are taking input from the user for row number, column number, first matrix elements and second matrix elements. Then we are performing multiplication on the matrices entered by the user.
In matrix multiplication first matrix one row element is multiplied by second matrix all column elements.
Write a c program to print multiplication of 2 matrices.
Input:
first matrix elements: 1 1 1 2 2 2 3 3 3
second matrix elements 1 1 1 2 2 2 3 3 3
Output:
multiplication of the matrix: 6 6 6 12 12 12 18 18 18
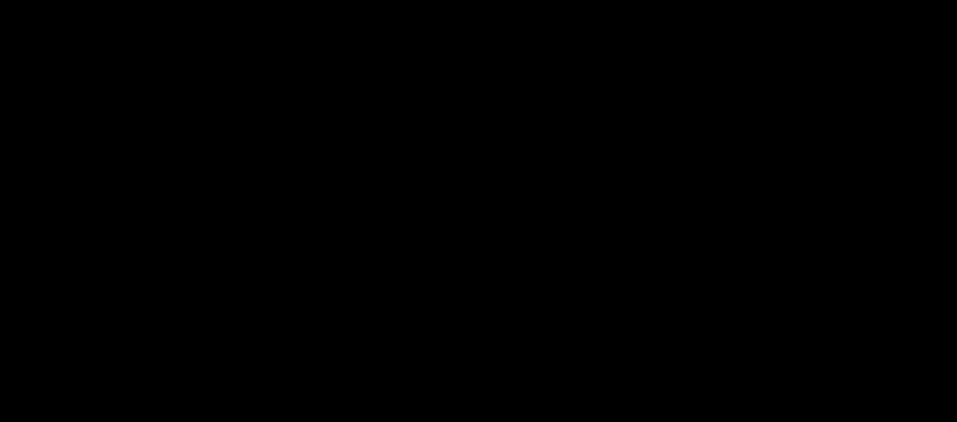
#include<stdio.h>
#include<stdlib.h>
int main(){
int a[10][10],b[10][10],mul[10][10],r,c,i,j,k;
system("cls");
printf("enter the number of row=");
scanf("%d",&r);
printf("enter the number of column=");
scanf("%d",&c);
printf("enter the first matrix element=\n");
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
scanf("%d",&a[i][j]);
}
}
printf("enter the second matrix element=\n");
for(i=0;i<r;i++)
{
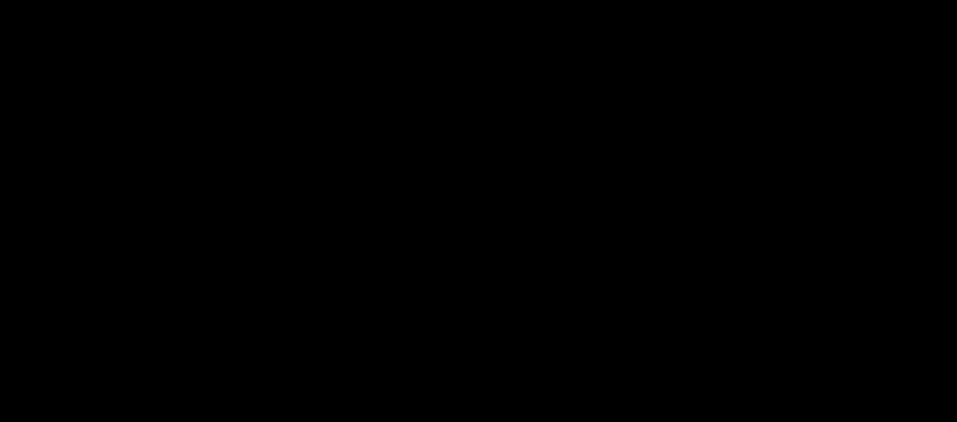
for(j=0;j<c;j++)
{
scanf("%d",&b[i][j]);
}
}
printf("multiply of the matrix=\n");
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
mul[i][j]=0;
for(k=0;k<c;k++)
{
mul[i][j]+=a[i][k]*b[k][j];
}
}
}
//for printing result
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
printf("%d\t",mul[i][j]);
}
printf("\n");
}
return 0;
}