5)Armstrong Number in Java
Let's write a java program to check whether the given number is armstrong number or not.
A positive number is called armstrong number if it is equal to the sum of cubes of its digits
For example 0, 1, 153, 370, 371, 407 etc.
Let's see the java program to check Armstrong Number.
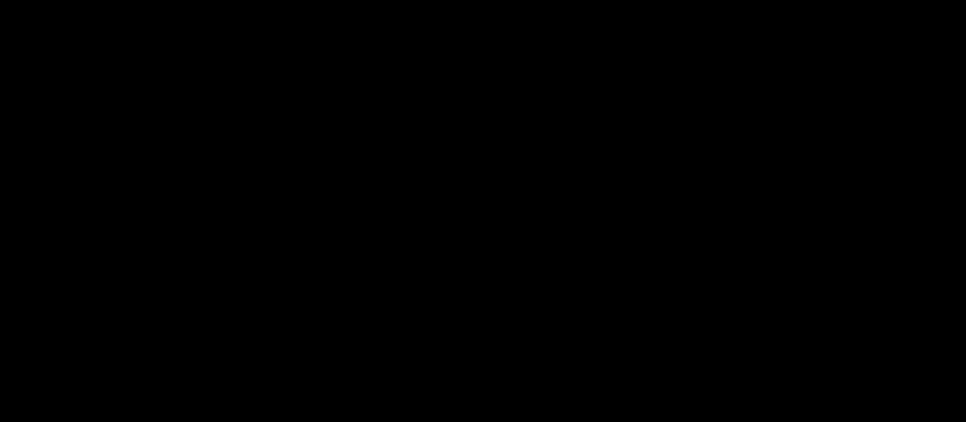
class ArmstrongExample{
public static void main(String[] args) {
int c=0,a,temp;
int n=153;//It is the number to check armstrong
temp=n;
while(n>0)
{
a=n%10;
n=n/10;
c=c+(a*a*a);
}
if(temp==c)
System.out.println("armstrong number");
else
System.out.println("Not armstrong number");
}
}
6) How to Generate Random Number in Java
In Java programming, we often required to generate random numbers while we develop applications. Many applications have the feature to generate numbers randomly, such as to verify the user many applications use the OTP. The best example of random numbers is dice. Because when we throw it, we get a random number between 1 to 6.
Random Number
Random numbers are the numbers that use a large set of numbers and selects a number using the mathematical algorithm. It satisfies the following two conditions:
-
The generated values uniformly distributed over a definite interval.
-
It is impossible to guess the future value based on current and past values.
Generating Random Number in Java
In Java, there is three-way to generate random numbers using the method and classes.
-
Using the random() Method
-
Using the Random Class
-
Using the ThreadLocalRandom Class
-
Using the ints() Method (in Java 8)
Using the Math.random() Method:
The Java Math class has many methods for different mathematical operations. One of them is the random() method. It is a static method of the Math class. We can invoke it directly. It generates only double type random number greater than or equal to 0.0 and less than 1.0. Before using the random() method, we must import the java.lang.Math class.
Syntax:
public static double random()
It does not accept any parameter. It returns a pseudorandom double that is greater than or equal to 0.0 and less than 1.0.
Remember: Every time we get a different output when we execute the program. Your output may differ from the output shown above.
We can also use the following formula if we want to a generate random number between a specified range.
Math.random() * (max - min + 1) + min
Let's create a program that generates random numbers using the random() method.
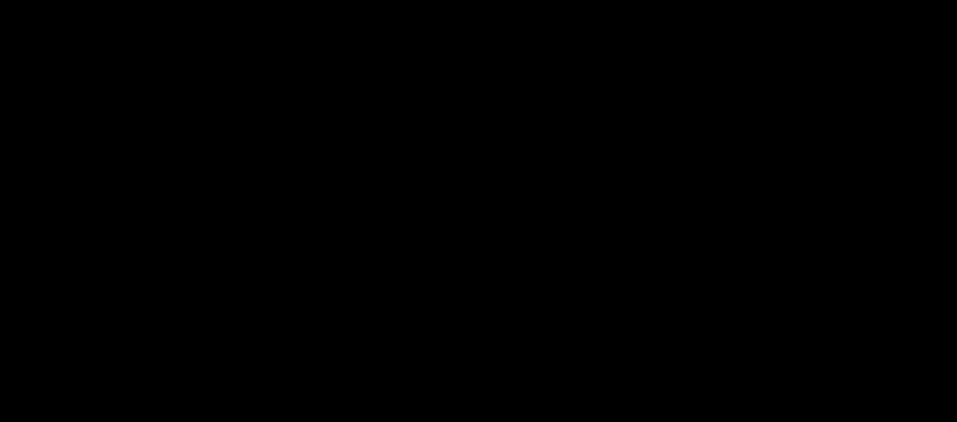
import java.lang.Math;
public class RandomNumberExample1
{
public static void main(String args[])
{
// Generating random numbers
System.out.println("1st Random Number: " + Math.random());
System.out.println("2nd Random Number: " + Math.random());
System.out.println("3rd Random Number: " + Math.random());
System.out.println("4th Random Number: " + Math.random());
}
}
Using the Random Class:
Another way to generate a random number is to use the Java Random class of the java.util package. It generates a stream of pseudorandom numbers. We can generate a random number of any data type, such as integer, float, double, Boolean, long. If you are going to use this class to generate random numbers, follow the steps given below:
-
First, import the class java.lang.Random.
-
Create an object of the Random class.
-
Invoke any of the following methods:
-
nextInt(int bound)
-
nextInt()
-
nextFloat()
-
nextDouble()
-
nextLong()
-
nextBoolean()
All the above methods return the next pseudorandom, homogeneously distributed value (corresponding method) from this random number generator's sequence. The nextDouble() and nextFloat() method generates random value between 0.0 and 1.0.
The nextInt(int bound) method accepts a parameter bound (upper) that must be positive. It generates a random number in the range 0 to bound-1.
Let's create a program that generates random numbers using the Random class.
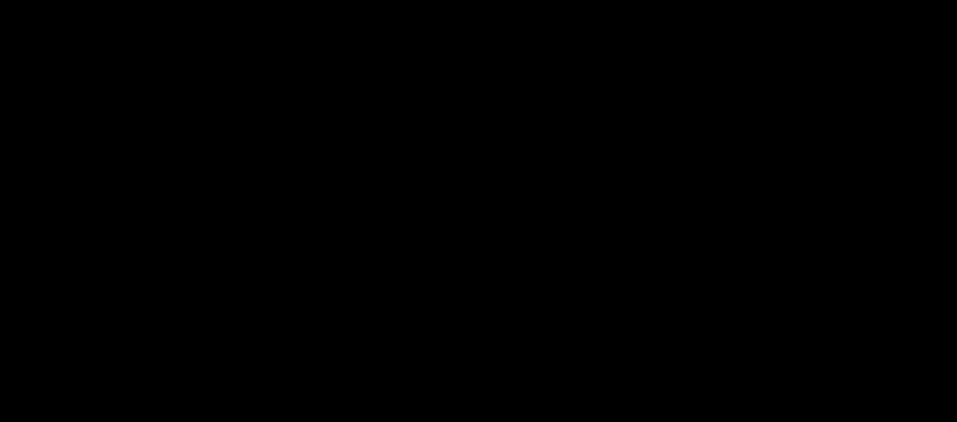
import java.util.Random;
public class RandomNumberExample3
{
public static void main(String args[])
{
// creating an object of Random class
Random random = new Random();
// Generates random integers 0 to 49
int x = random.nextInt(50);
// Generates random integers 0 to 999
int y = random.nextInt(1000);
// Prints random integer values
System.out.println("Randomly Generated Integers Values");
System.out.println(x);
System.out.println(y);
// Generates Random doubles values
double a = random.nextDouble();
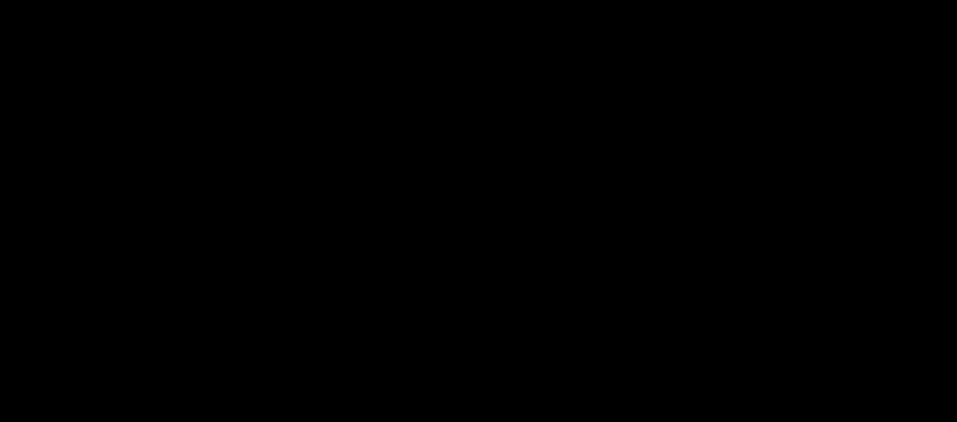
double b = random.nextDouble();
// Prints random double values
System.out.println("Randomly Generated Double Values");
System.out.println(a);
System.out.println(b);
// Generates Random float values
float f=random.nextFloat();
float i=random.nextFloat();
// Prints random float values
System.out.println("Randomly Generated Float Values");
System.out.println(f);
System.out.println(i);
// Generates Random Long values
long p = random.nextLong();
long q = random.nextLong();
// Prints random long values
System.out.println("Randomly Generated Long Values");
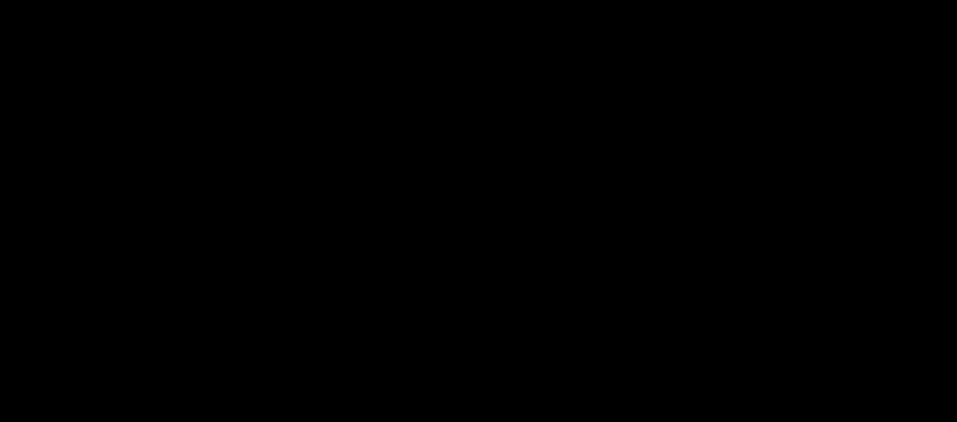
System.out.println(p);
System.out.println(q);
// Generates Random boolean values
boolean m=random.nextBoolean();
boolean n=random.nextBoolean();
// Prints random boolean values
System.out.println("Randomly Generated Boolean Values");
System.out.println(m);
System.out.println(n);
}
}