2) Prime Number Program in Java
Prime number in Java: Prime number is a number that is greater than 1 and divided by 1 or itself only. In other words, prime numbers can't be divided by other numbers than itself or 1.
For example 2, 3, 5, 7, 11, 13, 17.... are the prime numbers.
Let's see the prime number program in java. In this java program, we will take a number variable and check whether the number is prime or not.
Note: 0 and 1 are not prime numbers. The 2 is the only even prime number because all the other even numbers can be divided by 2.
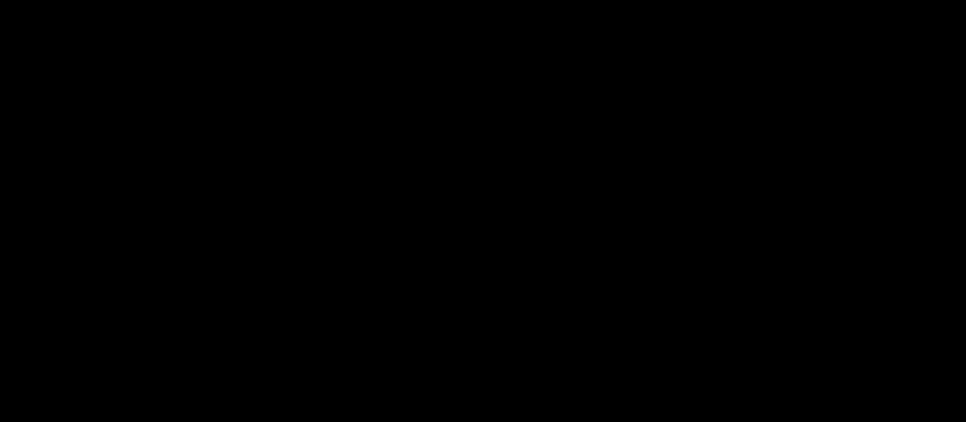
public class PrimeExample{
public static void main(String args[]){
int i,m=0,flag=0;
int n=3;//it is the number to be checked
m=n/2;
if(n==0||n==1){
System.out.println(n+" is not prime number");
}else{
for(i=2;i<=m;i++){
if(n%i==0){
System.out.println(n+" is not prime number");
flag=1;
break;
}
}
if(flag==0) { System.out.println(n+" is prime number"); }
}//end of else
}
}
3) Palindrome Program in Java
Palindrome number in java: A palindrome number is a number that is same after reverse. For example 545, 151, 34543, 343, 171, 48984 are the palindrome numbers. It can also be a string like LOL, MADAM etc.
Palindrome number algorithm
-
Get the number to check for palindrome
-
Hold the number in temporary variable
-
Reverse the number
-
Compare the temporary number with reversed number
-
If both numbers are same, print "palindrome number"
-
Else print "not palindrome number"
Let's see the palindrome program in java. In this java program, we will get a number variable and check whether number is palindrome or not.
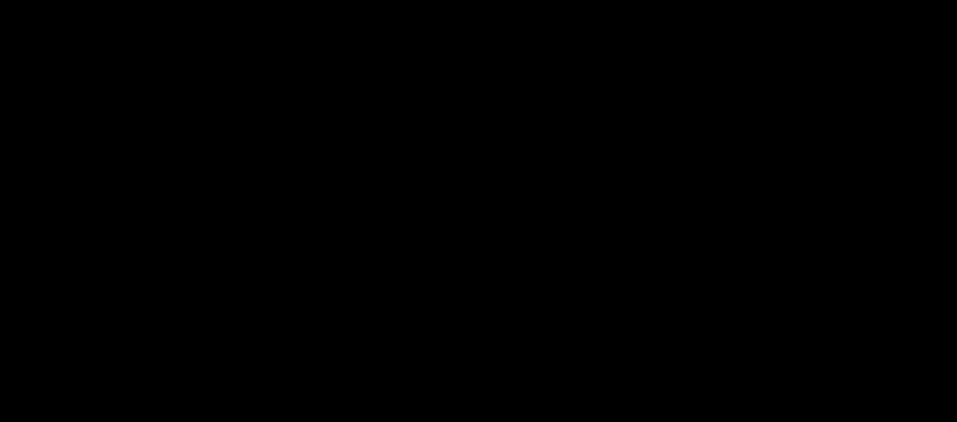
class PalindromeExample{
public static void main(String args[]){
int r,sum=0,temp;
int n=454;//It is the number variable to be checked for palindrome
temp=n;
while(n>0){
r=n%10; //getting remainder
sum=(sum*10)+r;
n=n/10;
}
if(temp==sum)
System.out.println("palindrome number ");
else
System.out.println("not palindrome");
}
}
4) Factorial Program in Java
Factorial of n is the product of all positive descending integers. Factorial of n is denoted by n!.
For example:
-
4! = 4*3*2*1 = 24
-
5! = 5*4*3*2*1 = 120
Here, 4! is pronounced as "4 factorial", it is also called "4 bang" or "4 shriek".
The factorial is normally used in Combinations and Permutations (mathematics).
There are many ways to write the factorial program in java language. Let's see the 2 ways to write the factorial program in java.
-
Factorial Program using loop
-
Factorial Program using recursion
Factorial Program using loop in java
Let's see the factorial Program using loop in java.
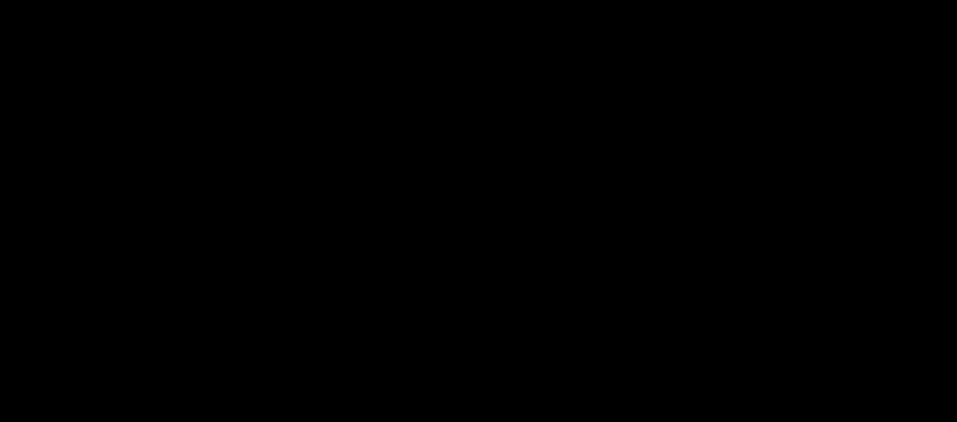
class FactorialExample{
public static void main(String args[]){
int i,fact=1;
int number=5;//It is the number to calculate factorial
for(i=1;i<=number;i++){
fact=fact*i;
}
System.out.println("Factorial of "+number+" is: "+fact);
}
}
Factorial Program using recursion in java
Let's see the factorial program in java using recursion.
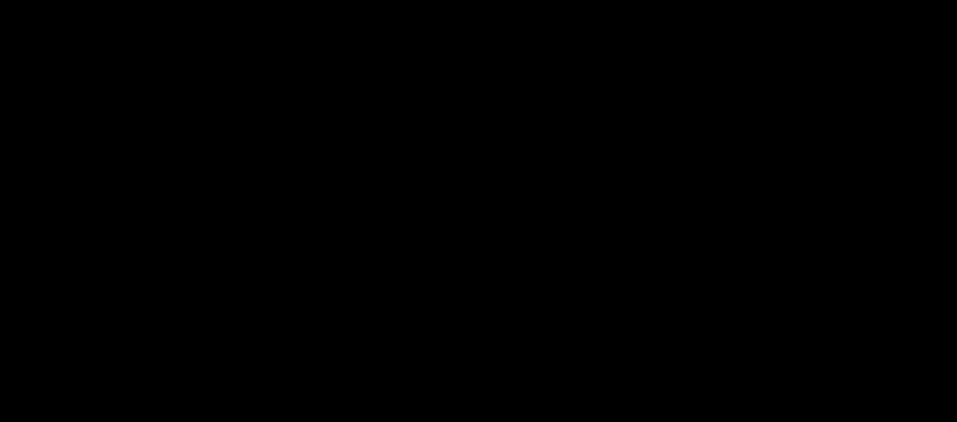
class FactorialExample2{
static int factorial(int n){
if (n == 0)
return 1;
else
return(n * factorial(n-1));
}
public static void main(String args[]){
int i,fact=1;
int number=4;//It is the number to calculate factorial
fact = factorial(number);
System.out.println("Factorial of "+number+" is: "+fact);
}
}