Basic Programs
1) Hello Python
This is the most basic Python program. It specifies how to print any statement in Python.
In old python (up to Python 2.7.0), print command is not written in parenthesis but in new python software (Python 3.4.3), it is mandatory to add a parenthesis to print a statement.

print ('Hello Python')
2) Python program to do arithmetical operations
The arithmetic operations are performed by calculator where we can perform addition, subtraction, multiplication and division. This example shows the basic arithmetic operations i.e.
-
Addition
-
Subtraction
-
Multiplication
-
Division
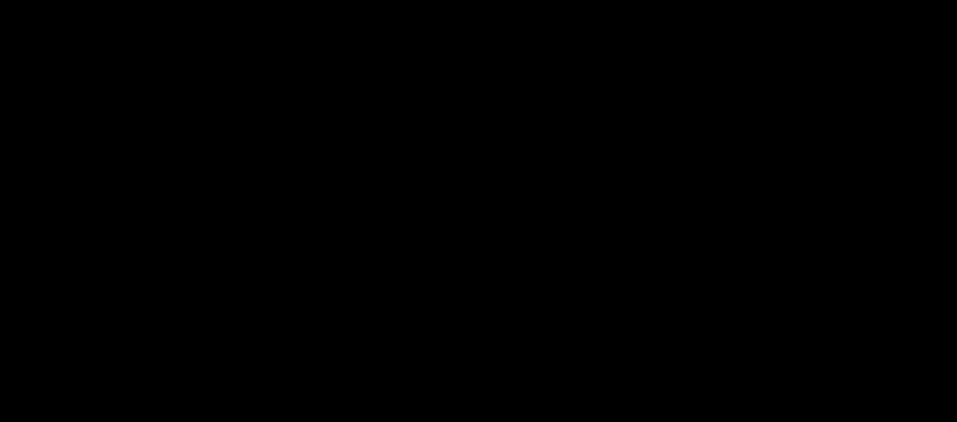
# Store input numbers:
num1 = input('Enter first number: ')
num2 = input('Enter second number: ')
# Add two numbers
sum = float(num1) + float(num2)
# Subtract two numbers
min = float(num1) - float(num2)
# Multiply two numbers
mul = float(num1) * float(num2)
#Divide two numbers
div = float(num1) / float(num2)
# Display the sum
print('The sum of {0} and {1} is {2}'.format(num1, num2, sum))
# Display the subtraction
print('The subtraction of {0} and {1} is {2}'.format(num1, num2, min))
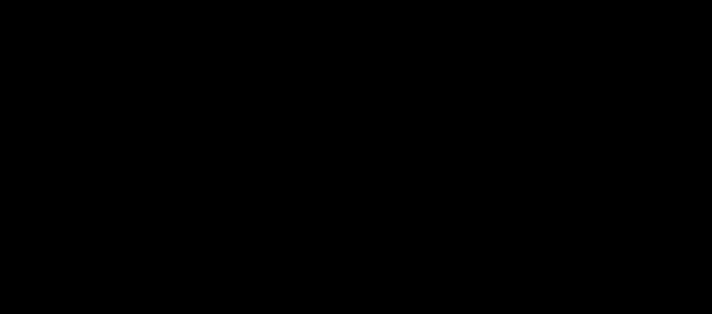
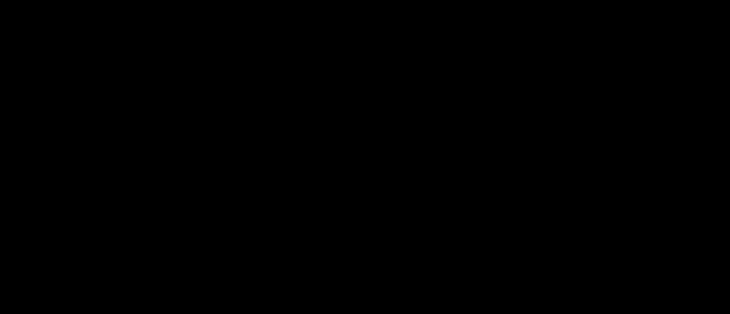
# Display the multiplication
print('The multiplication of {0} and {1} is {2}'.format(num1, num2, mul))
# Display the division
print('The division of {0} and {1} is {2}'.format(num1, num2, div))