3) Python program to find the area of a triangle
Mathematical formula:
Area of a triangle = (s*(s-a)*(s-b)*(s-c))-1/2
Here s is the semi-perimeter and a, b and c are three sides of the triangle.
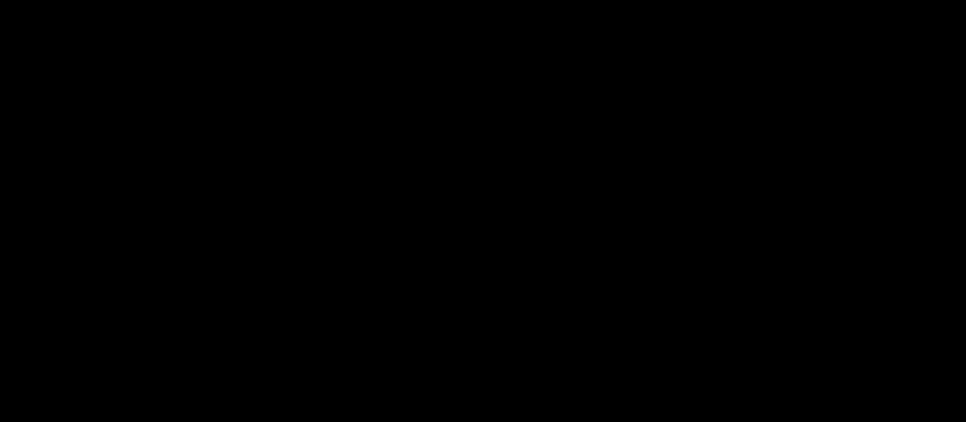
# Three sides of the triangle is a, b and c:
a = float(input('Enter first side: '))
b = float(input('Enter second side: '))
c = float(input('Enter third side: '))
# calculate the semi-perimeter
s = (a + b + c) / 2
# calculate the area
area = (s*(s-a)*(s-b)*(s-c)) ** 0.5
print('The area of the triangle is %0.2f' %area)
4) Python program to solve quadratic equation
Quadratic equation:
Quadratic equation is made from a Latin term "quadrates" which means square. It is a special type of equation having the form of:
ax2+bx+c=0
Here, "x" is unknown which you have to find and "a", "b", "c" specifies the numbers such that "a" is not equal to 0. If a = 0 then the equation becomes liner not quadratic anymore.
In the equation, a, b and c are called coefficients.
Let's take an example to solve the quadratic equation 8x2 + 16x + 8 = 0
See this example:
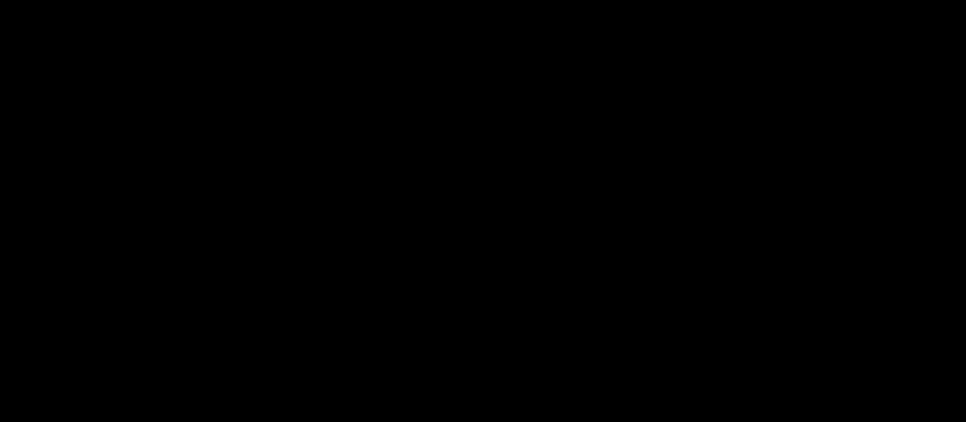
# import complex math module
import cmath
a = float(input('Enter a: '))
b = float(input('Enter b: '))
c = float(input('Enter c: '))
# calculate the discriminant
d = (b**2) - (4*a*c)
# find two solutions
sol1 = (-b-cmath.sqrt(d))/(2*a)
sol2 = (-b+cmath.sqrt(d))/(2*a)
print('The solution are {0} and {1}'.format(sol1,sol2))