7) Reverse Number
We can reverse a number in c using loop and arithmetic operators. In this program, we are getting number as input from the user and reversing that number.
Write a c program to reverse given number.
Input: 123
Output: 321
Let's see a simple c example to reverse a given number.
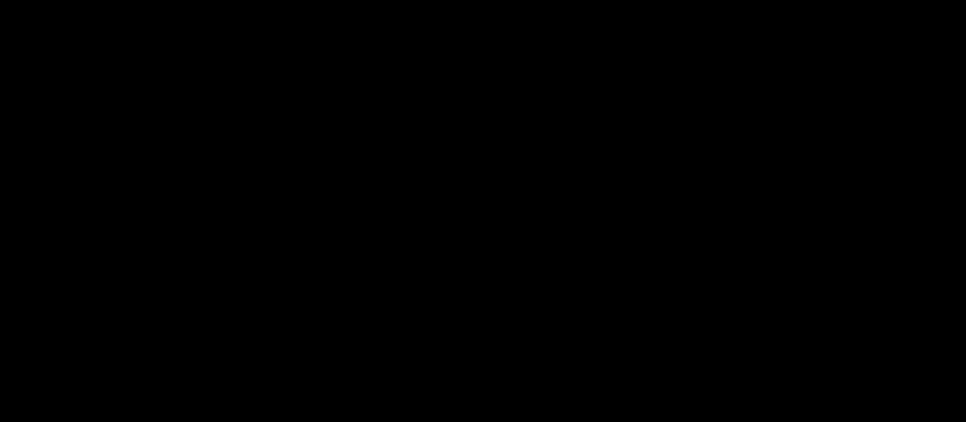
#include<stdio.h>
int main()
{
int n, reverse=0, rem;
printf("Enter a number: ");
scanf("%d", &n);
while(n!=0)
{
rem=n%10;
reverse=reverse*10+rem;
n/=10;
}
printf("Reversed Number: %d",reverse);
return 0;
}
8) Swap two numbers without using third variable
We can swap two numbers without using third variable. There are two common ways to swap two numbers without using third variable:
-
By + and -
-
By * and /
Write a c program to swap two numbers without using third variable.
Input: a=10 b=20
Output: a=20 b=10
Program 1: Using + and -
Let's see a simple c example to swap two numbers without using third variable.
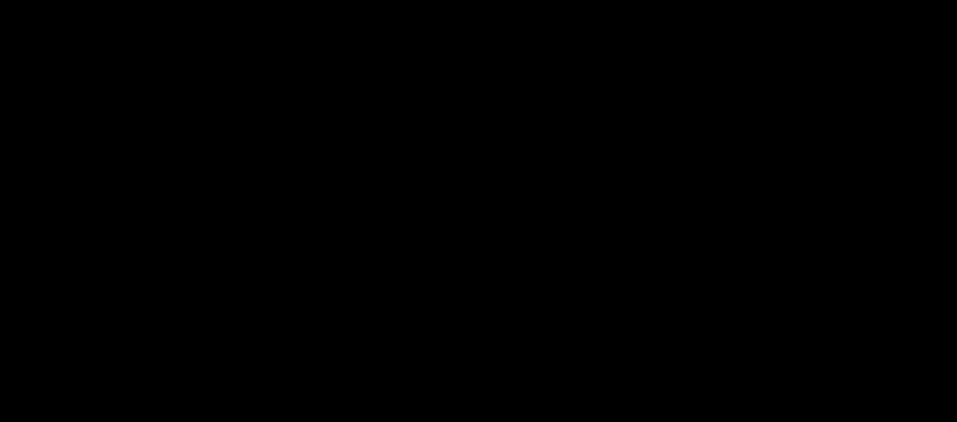
#include<stdio.h>
int main()
{
int a=10, b=20;
printf("Before swap a=%d b=%d",a,b);
a=a+b;//a=30 (10+20)
b=a-b;//b=10 (30-20)
a=a-b;//a=20 (30-10)
printf("\nAfter swap a=%d b=%d",a,b);
return 0;
}