5) Armstrong number
Before going to write the c program to check whether the number is Armstrong or not, let's understand what is Armstrong number.
Armstrong number is a number that is equal to the sum of cubes of its digits. For example 0, 1, 153, 370, 371 and 407 are the Armstrong numbers.
Write a c program to check armstrong number.
Input: 153
Output: armstrong
Input: 22
Output: not armstrong
Let's see the c program to check Armstrong Number in C
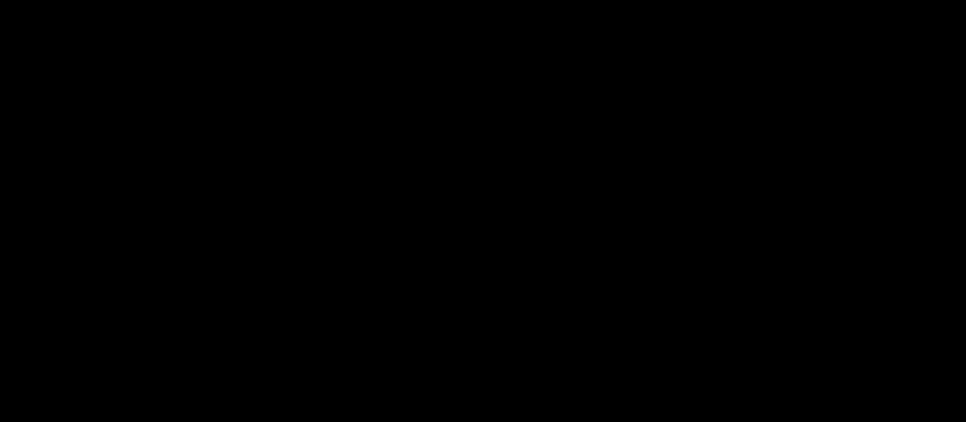
#include<stdio.h>
int main()
{
int n,r,sum=0,temp;
printf("enter the number=");
scanf("%d",&n);
temp=n;
while(n>0)
{
r=n%10;
sum=sum+(r*r*r);
n=n/10;
}
if(temp==sum)
printf("armstrong number ");
else
printf("not armstrong number");
return 0;
}
6) Sum of Digits
We can write the sum of digits program in c language by the help of loop and mathematical operation only.
Sum of digits algorithm
To get sum of each digits by c program, use the following algorithm:
-
Step 1: Get number by user
-
Step 2: Get the modulus/remainder of the number
-
Step 3: sum the remainder of the number
-
Step 4: Divide the number by 10
-
Step 5: Repeat the step 2 while number is greater than 0.
Write a c program to print sum of digits.
Input: 234
Output: 9
Input: 12345
Output: 15
Let's see the sum of digits program in C.
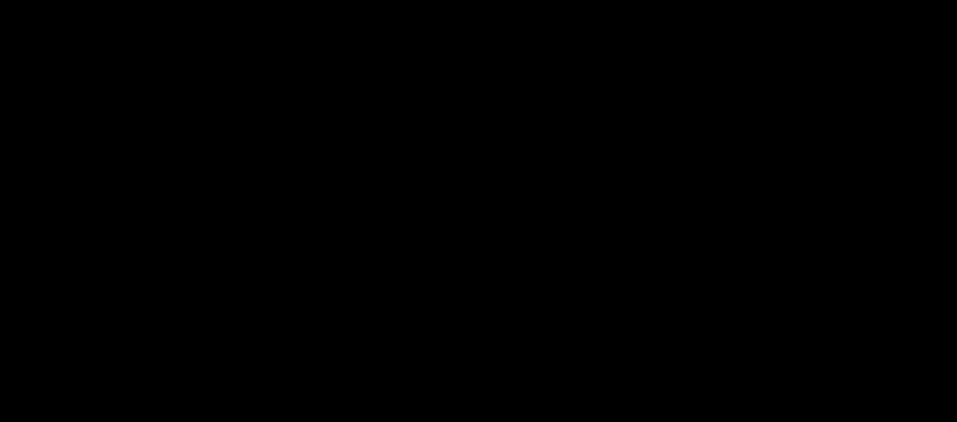
#include<stdio.h>
int main()
{
int n,sum=0,m;
printf("Enter a number:");
scanf("%d",&n);
while(n>0)
{
m=n%10;
sum=sum+m;
n=n/10;
}
printf("Sum is=%d",sum);
return 0;
}