4) Factorial
Factorial of n is the product of all positive descending integers. Factorial of n is denoted by n!.
There are many ways to write the factorial program in c++ language. Let's see the 2 ways to write the factorial program.
-
Factorial Program using loop
-
Factorial Program using recursion
Write a c++ program to print factorial of a number.
Input: 5
Output: 120
Input: 6
Output: 720
Factorial Program using loop
Let's see the factorial Program using loop.
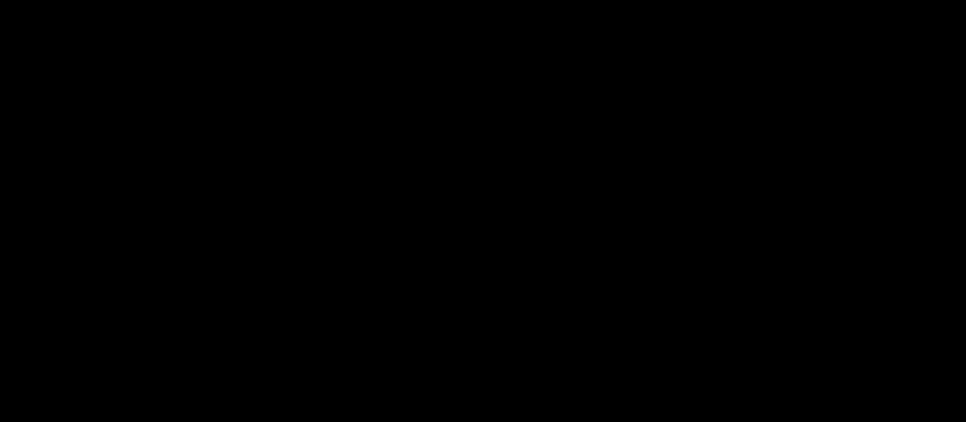
#include <iostream>
using namespace std;
int main()
{
int i,fact=1,number;
cout<<"Enter any Number: ";
cin>>number;
for(i=1;i<=number;i++){
fact=fact*i;
}
cout<<"Factorial of " <<number<<" is: "<<fact<<endl;
return 0;
}
Factorial Program using recursion in C++
Let's see the factorial program in c++ using recursion.
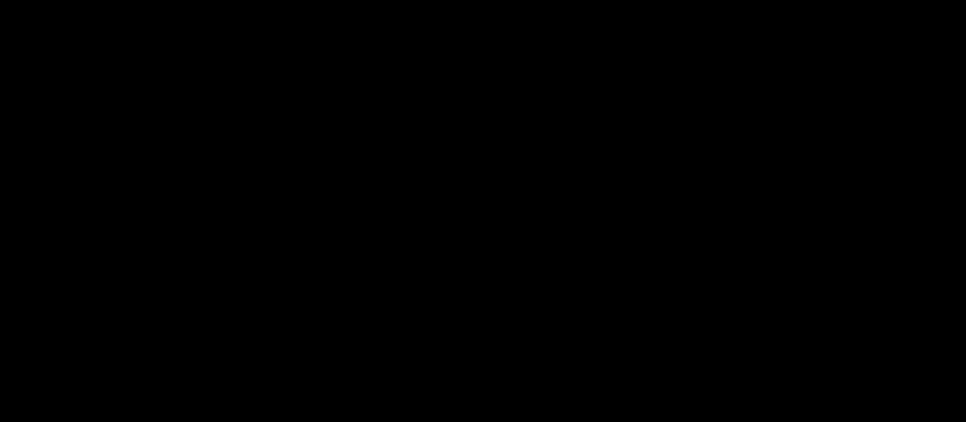
#include<iostream>
using namespace std;
int main()
{
int factorial(int);
int fact,value;
cout<<"Enter any number: ";
cin>>value;
fact=factorial(value);
cout<<"Factorial of a number is: "<<fact<<endl;
return 0;
}
int factorial(int n)
{
if(n<0)
return(-1); /*Wrong value*/
if(n==0)
return(1); /*Terminating condition*/
else
{
return(n*factorial(n-1));
}
}