2) Prime number
Prime number is a number that is greater than 1 and divided by 1 or itself. In other words, prime numbers can't be divided by other numbers than itself or 1. For example 2, 3, 5, 7, 11, 13, 17, 19, 23.... are the prime numbers.
Write a c++ program to check prime number.
Input: 17
Output: not prime number
Input: 57
Output: prime number
Note: Zero (0) and 1 are not considered as prime numbers. Two (2) is the only one even prime number because all the numbers can be divided by 2.
Let's see the prime number program in C++. In this c++ program, we will take an input from the user and check whether the number is prime or not.

#include <iostream>
using namespace std;
int main()
{
int n, i, m=0, flag=0;
cout << "Enter the Number to check Prime: ";
cin >> n;
m=n/2;
for(i = 2; i <= m; i++)
{
if(n % i == 0)
{
cout<<"Number is not Prime."<<endl;
flag=1;
break;
}
}
if (flag==0)
cout << "Number is Prime."<<endl;
return 0;
}
3) Palindrome number
A palindrome number is a number that is same after reverse. For example 121, 34543, 343, 131, 48984 are the palindrome numbers.
Palindrome number algorithm
-
Get the number from user
-
Hold the number in temporary variable
-
Reverse the number
-
Compare the temporary number with reversed number
-
If both numbers are same, print palindrome number
-
Else print not palindrome number
Write a c++ program to check palindrome number.
Input: 121
Output: not palindrome number
Input: 113
Output: palindrome number
Let's see the palindrome program in C++. In this c++ program, we will get an input from the user and check whether number is palindrome or not.
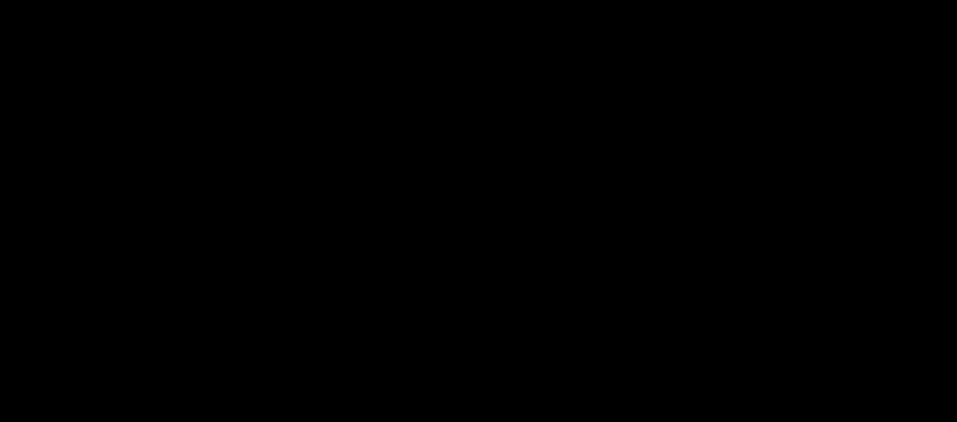
#include <iostream>
using namespace std;
int main()
{
int n,r,sum=0,temp;
cout<<"Enter the Number=";
cin>>n;
temp=n;
while(n>0)
{
r=n%10;
sum=(sum*10)+r;
n=n/10;
}
if(temp==sum)
cout<<"Number is Palindrome.";
else
cout<<"Number is not Palindrome.";
return 0;
}