7) Python program to convert kilometers to miles
Here, we are going to see the python program to convert kilometers to miles. Let's understand kilometers and miles first.
Kilometer:
The kilometer is a unit of length in the metric system. It is equivalent to 1000 meters.
Miles:
Mile is also the unit of length. It is equal to 1760 yards.
Conversion formula:
1 kilometer is equal to 0.62137 miles.
-
Miles = kilometer * 0.62137
-
Kilometer = Miles / 0.62137
See this example:
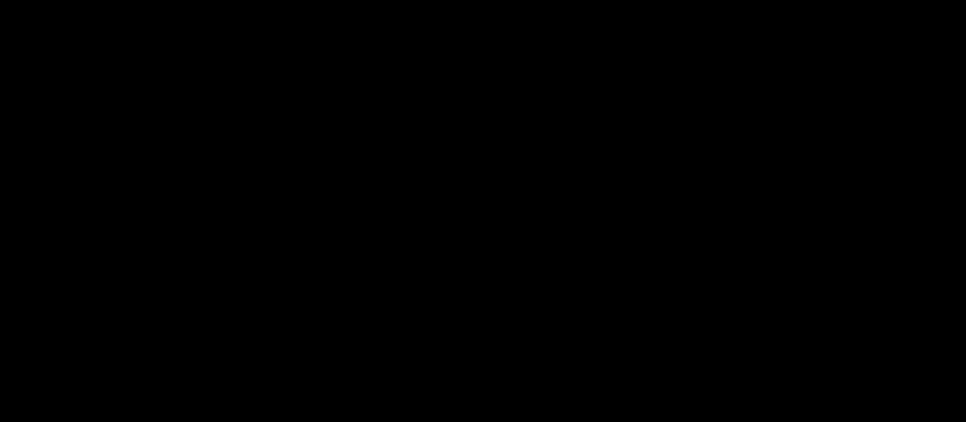
# Collect input from the user
kilometers = float(input('How many kilometers?: '))
# conversion factor
conv_fac = 0.621371
# calculate miles
miles = kilometers * conv_fac
print('%0.3f kilometers is equal to %0.3f miles' %(kilometers,miles))
8) Python program to convert Celsius to Fahrenheit
Celsius:
Celsius is a unit of measurement for temperature. It is also known as centigrade. It is a SI derived unit used by most of the countries worldwide.
It is named after the Swedish astronomer Anders Celsius.
Fahrenheit:
Fahrenheit is also a temperature scale. It is named on Polish-born German physicist Daniel Gabriel Fahrenheit. It uses degree Fahrenheit as a unit for temperature.
Conversion formula:
T(℉) = T(℃) x 9/5 + 32
Or,
T(℉) = T(℃) x 1.8 + 32
See this example:
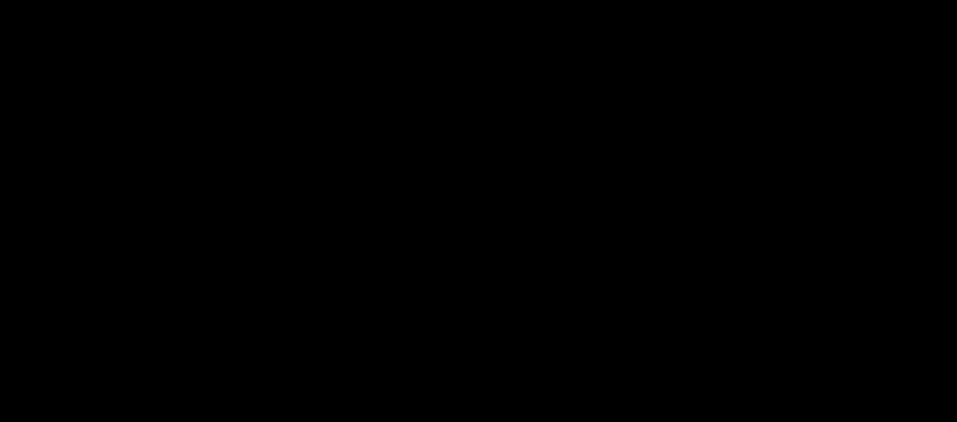
# Collect input from the user
celsius = float(input('Enter temperature in Celsius: '))
# calculate temperature in Fahrenheit
fahrenheit = (celsius * 1.8) + 32
print('%0.1f Celsius is equal to %0.1f degree Fahrenheit'%(celsius,fahrenheit))