Programs With Loops & Conditions
1) Python Program to check if a Number is Positive, Negative or Zero
We can use a Python program to distinguish that if a number is positive, negative or zero.
Positive Numbers: A number is known as a positive number if it has a greater value than zero. i.e. 1, 2, 3, 4 etc.
Negative Numbers: A number is known as a negative number if it has a lesser value than zero. i.e. -1, -2, -3, -4 etc.
See this example:

num = float(input("Enter a number: "))
if num > 0:
print("{0} is a positive number".format(num))
elif num == 0:
print("{0} is zero".format(num))
else:
print("{0} is negative number".format(num))
2) Python Program to Check if a Number is Odd or Even
Odd and Even numbers:
If you divide a number by 2 and it gives a remainder of 0 then it is known as even number, otherwise an odd number.
Even number examples: 2, 4, 6, 8, 10, etc.
Odd number examples:1, 3, 5, 7, 9 etc.
See this example:
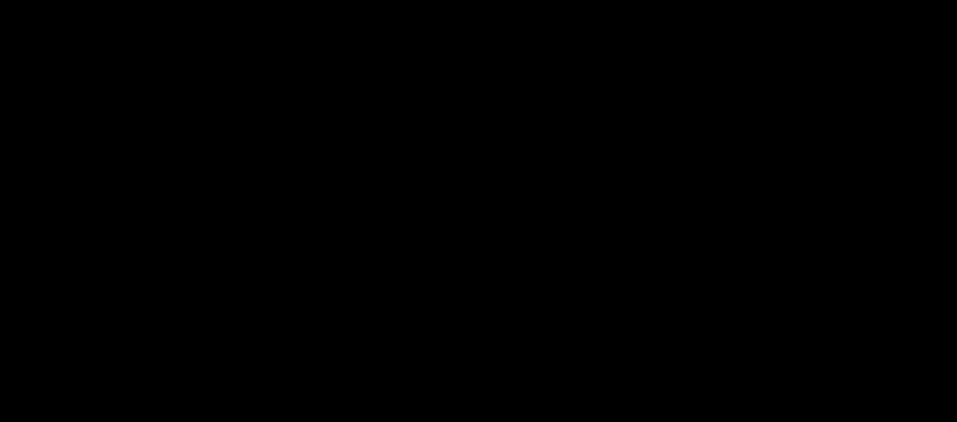
num = int(input("Enter a number: "))
if (num % 2) == 0:
print("{0} is Even number".format(num))
else:
print("{0} is Odd number".format(num))