3) Python Program to Transpose a Matrix
Transpose Matrix:
If you change the rows of a matrix with the column of the same matrix, it is known as transpose of a matrix. It is denoted as X'.
For example: The element at ith row and jth column in X will be placed at jth row and ith column in X'.
Let's take a matrix X, having the following elements:
For example X = [[1, 2], [3, 4], [5, 6]] would represent a 3x2 matrix. First row can be selected as X[0] and the element in first row, first column can be selected as X[0][0].
Let's take two matrices X and Y, having the following value:
X = [[1,2],
[4,5],
[7,8]]
See this example:

# iterate through rows
for i in range(len(X)):
for j in range(len(X[0])):
result[j][i] = X[i][j]
for r in result:
print(r)
4) Python Program to Sort Words in Alphabetic Order
Sorting:
Sorting is a process of arrangement. It arranges data systematically in a particular format. It follows some algorithm to sort data.
See this example:
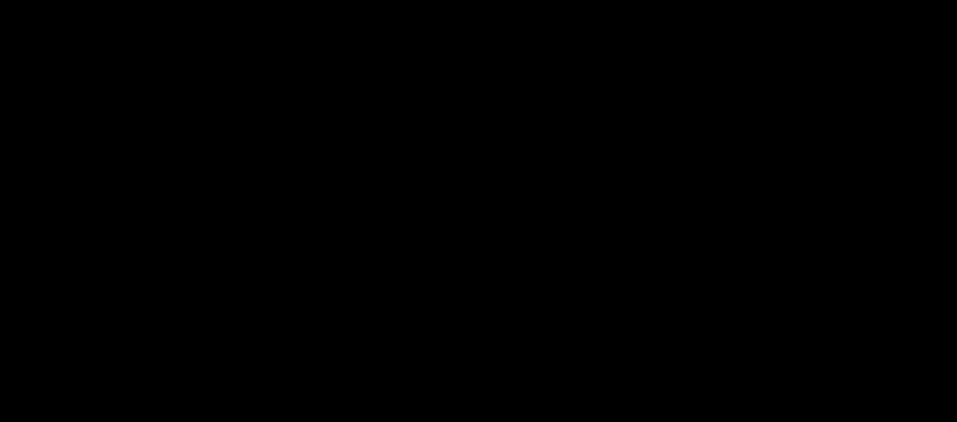
my_str = input("Enter a string: ")
# breakdown the string into a list of words
words = my_str.split()
# sort the list
words.sort()
# display the sorted words
for word in words:
print(word)